概要
コントローラーとビューの準備によって、プロジェクトの枠組みをつくった。
ここでは、仮置きのページとして3つのhtml.erbファイルの内容を書いていき、それらにスタイルを定義する。
掲示板の第1段階へ
ルーティング
第1段階では、それぞれのページのURLを指定して表示させる設定。
|
Rails.application.routes.draw do get '/top', to: 'pages#top' get '/sign_up', to: 'users#sign_up' get '/sign_in', to: 'users#sign_in' end |
ルーティングの状況を確認する。後でヘルパーのリンク先URLを指定するとき、Prefix_pathという定型句で指定することができる。
|
[vagrant@vagrant ex_bbs]$ rails routes Prefix Verb URI Pattern Controller#Action top GET /top(.:format) pages#top sign_up GET /sign_up(.:format) users#sign_up sign_in GET /sign_in(.:format) users#sign_in |
共通レイアウト
サインアウト中ページ用
application_signed_out.html.erb
サインアウト中のページには特にヘッダーメニューなどを表示しないので、デフォルトで生成されたapplication.html.erb
の内容通り。
ただしこちらを利用するのがsign_in
、sign_up
の2つのアクションだけと限定的なので、ファイル名を変更する。
|
<!DOCTYPE html> <html> <head> <title>ExBbs</title> <%= csrf_meta_tags %> <%= stylesheet_link_tag 'application', media: 'all', 'data-turbolinks-track': 'reload' %> <%= javascript_include_tag 'application', 'data-turbolinks-track': 'reload' %> </head> <body> <%= yield %> </body> </html> |
users_controller.rb
デフォルトのapplication.html.erb以外を共通レイアウトとして使う場合は、コントローラーから明示的に呼び出す。
|
class UsersController < ApplicationController def sign_up render layout: 'application_signed_out' end def sign_in render layout: 'application_signed_out' end end |
サインイン済みページ用
application.html.erb
サイン済みユーザーに対しては、第1段階ではtop
ページのみ表示させるが、今後いろいろな機能をつけていく。こちらの方が主になるので、ファイル名をデフォルトのapplication.html.erb
とし、ヘッダーメニューを加える。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
|
<!DOCTYPE html> <html> <head> <title>ExBbs</title> <%= csrf_meta_tags %> <%= stylesheet_link_tag 'application', media: 'all', 'data-turbolinks-track': 'reload' %> <%= javascript_include_tag 'application', 'data-turbolinks-track': 'reload' %> </head> <body> <header> <div class="header_logo"></div> <h1>EX-BBS</h1> <ul class="header_menu"> <li><%= link_to("投稿する", "#") %></li> <li><%= link_to("プロフィール", "#") %></li> <li><%= link_to("サインアウト", "#") %></li> </ul> </header> <%= yield %> </body> </html> |
header.scss
ヘッダーに対するスタイル定義(HTML/CSSのヘッダーメニューを参照)。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
|
:root { --header-height: 50px; --foreground-color: #00008b; } header { display: flex; align-items: center; justify-content: space-between; height: var(--header-height); background-color: #cff; .header_logo { background-image: url("logo.png"); background-repeat: no-repeat; background-size: contain; width: calc(var(--header-height) * 2); height: var(--header-height); } h1 { font-size: 32px; color: var(--foreground-color); } .header_menu { display: flex; list-style: none; & li { display: flex; align-items: center; justify-content: center; margin: 10px 0px 10px 5px; width: 100px; font-size: 14px; color: var(--foreground-color); & a { text-decoration: none; color: var(--foreground-color); } & + li { border-left: 1px solid var(--foreground-color); } } } } |
個別ページ
topページ
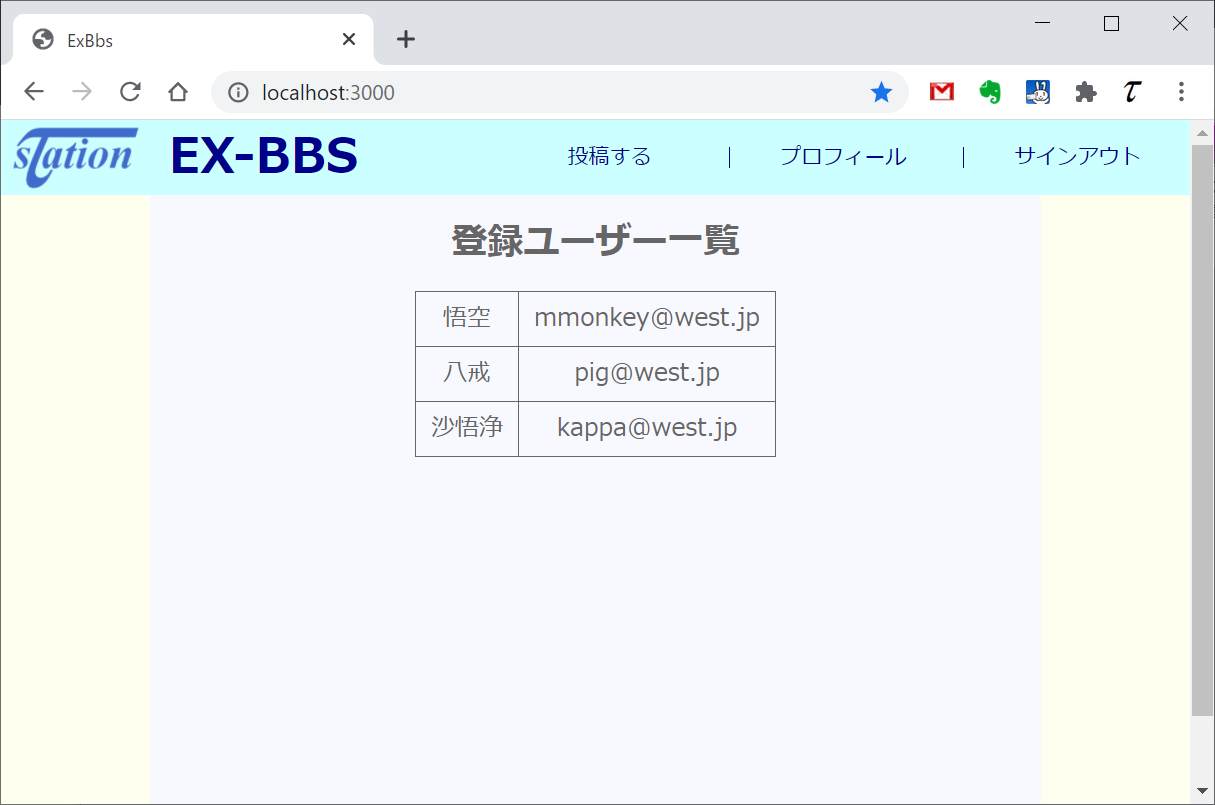
top.html
第1段階では、仮置きとしてユーザー一覧を表示させる。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
|
<div class="top_page"> <h2>登録ユーザー一覧</h2> <div class="user_list"> <table> <tr> <td>悟空</td> <td>mmonkey@taustation.jp</td> </tr> <tr> <td>八戒</td> <td>pig@taustation.jp</td> </tr> <tr> <td>沙悟浄</td> <td>kappa@taustation.jp</td> </tr> </table> </div> </main> |
pages.scss
top
ページに対するスタイル定義は、topアクション
を持つpages
コントローラーと同名のscssに書く。
メイン部分の領域が縦一杯に広がるようにするために、html
とbody
のheight
に100%を指定している。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
|
html { height: 100%; } body { background: #eee; height: 100%; } .top_page { --text-color: #666; width: 75%; height: 100%; margin: auto; background-color: #f8f8ff; text-align: center; h2 { padding: 20px 0; font-size: 24px; color: var(--text-color); } .user_list { display: inline-block; color: #666; td { border: 1px solid var(--text-color); align: center; padding: 10px; color: var(--text-color); } } } |
sign_upページ・sign_inページ
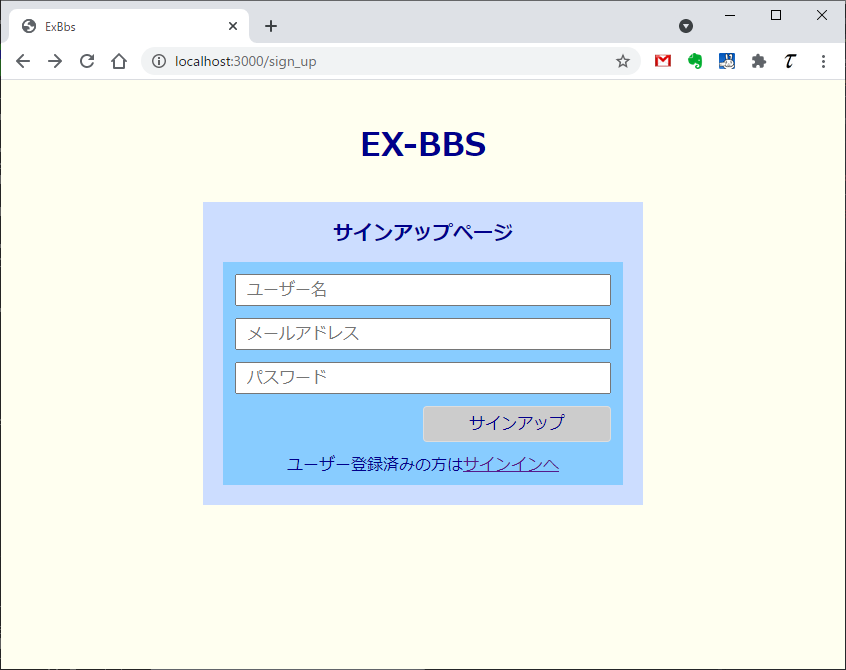
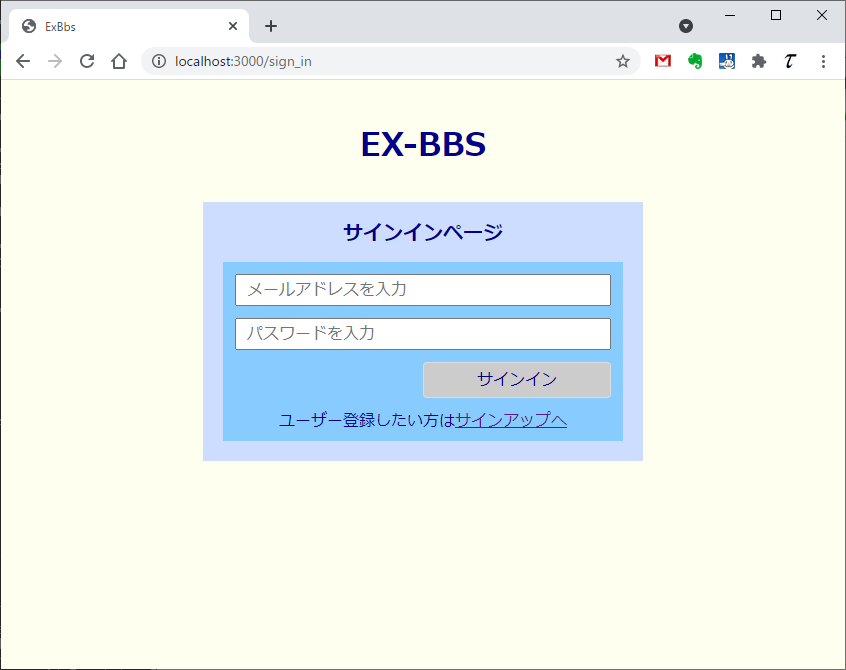
sign_up.html.erb
sign_up
とsign_in
は殆ど似たような内容・デザイン。モデルなしのform_with
ヘルパーを使い、URLは仮置きしている。
また、link_to
ヘルパーのリンク先に、ルーティングのPrefixを使った名前でURLを指定している。
|
<div class="sign_up_page"> <h1>EX-BBS</h1> <div class="sign_up_form"> <h2>サインアップページ</h2> <%= form_with url: "#", local: true do |f| %> <%= f.text_field :name, placeholder: "ユーザー名" %> <%= f.email_field :email, placeholder: "メールアドレス" %> <%= f.password_field :password, placeholder: "パスワード" %> <button>サインアップ</button> <p>ユーザー登録済みの方は<%= link_to "サインインへ", sign_in_path %></p> <% end %> </div> </div> |
sign_in.thml.erb
|
<div class="sign_in_page"> <h1>EX-BBS</h1> <div class="sign_in_form"> <h2>サインインページ</h2> <%= form_with url: sign_in_path, local: true do |f| %> <%= f.email_field :email, placeholder: "メールアドレスを入力" %> <%= f.password_field :password, placeholder: "パスワードを入力" %> <button>サインイン</button> <p>ユーザー登録したい方は<%= link_to "サインアップへ", sign_up_path %></p> <% end %> </div> </div> |
users.scss
sign_up
とsign_in
の2つのビューのスタイルは、それらのアクションを持つusers
コントローラーと同名のusers.scss
に書く。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66
|
body { background-color: #fffff0; } .sign_in_page, .sign_up_page { overflow-y: hidden; // サインイン/サインアップページの表題スタイル h1 { width: 300px; margin: 50px auto 0 auto; font-size: 32px; text-align: center; color: var(--title-color) } // フォームブロックのスタイル .sign_in_form, .sign_up_form { margin: 40px auto 0 auto; width: 400px; padding: 20px; background-color: #cdf; // フォームブロックの表題スタイル h2 { margin-bottom: 20px; font-family: fantasy; font-size: 20px; text-align: center; color: var(--title-color) } // フォーム本体 form { padding: 12px; background-color: #8cf; // フォーム内のinput要素 input { display: block; box-sizing: border-box; width: 100%; height: 32px; margin-bottom: 12px; padding: 3px 10px; font-size: 16px; } // フォーム内の送信ボタン button { display: block; width: 50%; margin-left: auto; margin-bottom: 15px; padding: 5px; background-color: #ccc; border: 1px solid #ddd; border-radius: 4px; font-size: 16px; color: var(--title-color); &:hover { background-color: #d0d0d0; } } // リンク関連の色 p { text-align: center; color: var(--title-color) } } } } |
リセットCSS
reset.scss
リセットCSSにはEric Meyer’s “Reset CSS” 2.0を使った。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
|
/* html5doctor.com Reset Stylesheet v1.6.1 Last Updated: 2010-09-17 Author: Richard Clark - http://richclarkdesign.com Twitter: @rich_clark */ html, body, div, span, object, iframe, h1, h2, h3, h4, h5, h6, p, blockquote, pre, abbr, address, cite, code, del, dfn, em, img, ins, kbd, q, samp, small, strong, sub, sup, var, b, i, dl, dt, dd, ol, ul, li, fieldset, form, label, legend, table, caption, tbody, tfoot, thead, tr, th, td, article, aside, canvas, details, figcaption, figure, footer, header, hgroup, menu, nav, section, summary, time, mark, audio, video { margin:0; padding:0; border:0; outline:0; font-size:100%; vertical-align:baseline; background:transparent; } ..... |
スタイルの読み込み
application.html.erb
、application_signed_out.html.erb
のいずれも、最初にapplication.css
を呼び出している。その中で必要なSCSSファイルを読み込んでいる。
書くスタイルファイルが読み込まれるのは1度だけなので、最初にreset.scss
を読み込み、その後require_tree
でそれ以外のすべてのファイルを読み込む。
|
*= require reset.scss *= require_tree . |